Typescript is an open-source programming language by Microsoft. Typescript is a statically typed language like C, C++, and Java. And Typescript makes javascript more capable. However, Javascript is a dynamically typed language i.e Checking the type of variable, object, function, etc is not mandatory. But We can enable static typing and perform type checks using TypeScript.
Table Of Contents
Introduction to TypeScript
- Let's declare a variable
age
and assign a value to theage
variable. Consider the following example without TypeScript
let age = 25
- In the above example, We didn't perform a type check. However javascript dynamically assigned the type
string
to the variableage
. We can check the type of age using thetypeof
property as shown below:
console.log(typeof age)
- Now, We will write the same example using typescript. We will declare a variable
age
and perform a type check as shown below:
let age: number = '25'
- We will run the above example using TypeScript Playground and get the following output
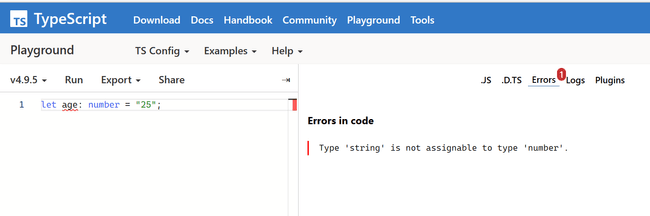
- In the above output, There is an error showing:
Type 'string' is not assignable to the type 'number'
. Hence, TypeScript is checking the type of theage
variable before executing the code. - Now, We will assign a value of type
number
to the variableage
and fix the error as shown below
let age: number = 25
Starting a Node JS Project
- Open the CMD (Command Line Tool) and create the folder
node-js-with-typescript
using the following command:
mkdir node-js-with-typescript
- Go to the current folder
node-js-with-typescript
and initialize a node project with the following commands:
cd node-js-with-typescript
npm init -y
- Next, Use the following command to open
node-js-with-typescript
folder inVisual Studio Code
.
code .
Note: You can use any code editor to work with the nodejs project. However, It is recommended to use Visual Studio Code
for this article.
- Now, the current folder structure looks like this:
node-js-with-typescript/
└─ package.json
Installing and Configuring TypeScript
- Node JS doesn't provide built-in support to use typescript. We have to add the npm package
typescript
to the node js application using the following command:
npm install -g typescript
- We can check the installed version of
typescript
as shown below:
tsc --version
- Now, We will initiate a TypeScript project for the Node JS Application
tsc --init
- The above command will create a file
tsconfig.json
inside the foldernode-js-with-typescript
- Replace the code of
tsconfig.json
with the following code block:
{
"compilerOptions": {
"outDir": "./dist",
/* Language and Environment */
"target": "es2016" /* Set the JavaScript language version for emitted JavaScript and include compatible library declarations. */,
/* Modules */
"module": "commonjs",
/* Allow 'import x from y' when a module doesn't have a default export. */
"esModuleInterop": true,
/* Type Checking */
"strict": true
}
}
- We will also Install typescript as a dev dependency using the following command:
npm install typescript --save-dev
- Create a new folder named
src
inside the foldernode-js-with-typescript
and a fileindex.ts
inside the foldersrc
. - Now, the current folder structure will look similar as shown below:
node-js-with-typescript/
├─ src
| ├─ index.ts
└─ package.json
- Add the following code block to the
index.ts
file:
function addTwoNumbers(x: number, y: number) {
let z = x + y
return z
}
- Compile the typescript files into javascript files using the following command:
npx tsc
- The above command will create a folder
dist
and a fileindex.js
inside the folderdist
. Now, We will run the following command:
node dist/index.js
Using ts-node
For Executing TypeScript Files
- Compiling typescript files and executing javascript separately adds a little headache. So, We use the npm package
ts-node
to execute typescript files directly - Install
ts-node
as a dev dependency with the following command:
npm install ts-node --save-dev
- Now, Run the following command to execute the
index.ts
file directly
npx ts-node src/index.ts
- Alternatively, We can add
start
property toscripts
object inpackage.json
as shown below:
"start": "ts-node src/index.ts"
- Now, We can simply use the command
npm run start
to execute typescript files.
Using TypeScript With NPM Packages
We can use typescript with any npm package. Here, We will learn to use typescript with Express JS.
Setting Up TypeScript for Express
- Open CMD(Command Line Tool) and run the following commands:
mkdir node-js-express-with-typescript
cd node-js-express-with-typescript
- Initialize a node project using the command:
npm init -y
- The above command will create a file
package.json
inside the foldernode-js-express-with-typescript
package.json file
- Install the npm package
express
with the following command:
npm install express --save
- Now, We will Install
typescript
to use TypeScript in Node JS Application,@types/node
to add type support for Node JS,@types/express
to add type support for Express JS,ts-node
to execute typescript files andnodemon
to restart the server if any changes occur to the file. - Install all the packages mentioned above with the following command:
npm install typescript @types/node @types/express ts-node nodemon --save-dev
- Open the folder
node-js-express-with-typescript
using the command:
code .
- Create a file
server.ts
inside the foldernode-js-express-with-typescript
and Add astart
property to thescripts
object in thepackage.json
file as shown below
"start": "nodemon src/server.ts",
Creating Express Server with TypeScript
- Add the following code to the
server.ts
file:
import express, { Request, Response, Application } from 'express'
// Initiate the express
const initExpress: Application = express()
initExpress.get('/', (req: Request, res: Response) => {
res.send(
`<h1>Hey! You successfully set up the express server with typescript.</h1>`,
)
})
// Listening to the express server at Port 9000
const PORT = 9000
initExpress.listen(PORT, () => {
console.log(`I am listening at ${PORT}`)
})
- In the above example,
Application
is the type forinitExpress
,Request
is the type forreq
andResponse
is the type forres
.
Prepare 'build' for Production
- Add the
build
property toscripts
object in thepackage.json
file as shown below:
"build": "npx tsc && node dist/index.js"
- Now, We can run the command
npm run build
to compile the typescript files and execute the javascript files.
Conclusion
- TypeScript is like the superpower of JavaScript that helps to reduce bugs in Node JS Applications.
- We can install TypeScript using the command
npm install typescript --save-dev
. - We can check the typescript version using command
tsc --version
and initiate typescript project using the commandtsc --init
. - We can compile typescript files to javascript files using the command
npx tsc
. @types/node
helps to add typescript support for Node JS. We can install@types/node
using the commandnpm install @types/node --save-dev
.@types/express
helps to add typescript support for Express JS. We can install@types/express
using the commandnpm install @types/express --save-dev
.